How to make a Telegram bot for sending error messages.
This guide outlines how to create a Telegram bot for sending error messages quickly. It involves creating and registering a bot with @BotFather, identifying the user's chatID with @myidbot, and writing Python code for sending messages with the requests library.
I'm often troubleshooting errors in my apps, so I need to be aware of any issues that come up quickly. I've been using the Mailgun API to send out error messages and related data, but that's not as speedy as having an instant message on my phone.
What do we need:
- Create and register new bot on telegram.
- Identify our telegram ChatID (telegram user account id).
- Write python code for sending messages.
Create and register new bot on telegram.
In this step we are going to use help of existing bot called @BotFather. Lookup @BotFather account in telegram search. Then click ‘start’ for conversation. It should look similar like on image below:
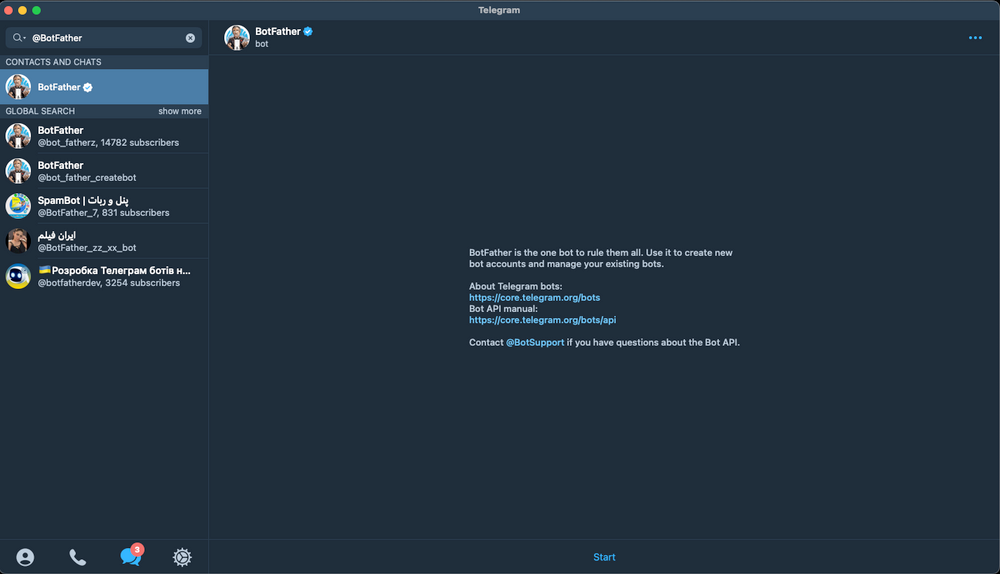
Type in "/newbot" in the prompt and hit enter. @BotFather will then ask for a name for your bot. Put in the name you want, and then you'll be asked for a username for your bot. Remember that the username has to end with 'bot'. I tried "error_bot", but it was already taken, so I tried something more unique - "ravnerr_bot" - and that was accepted. @BotFather will then provide you with an access token - keep it safe, as we'll need it in our Python code later.
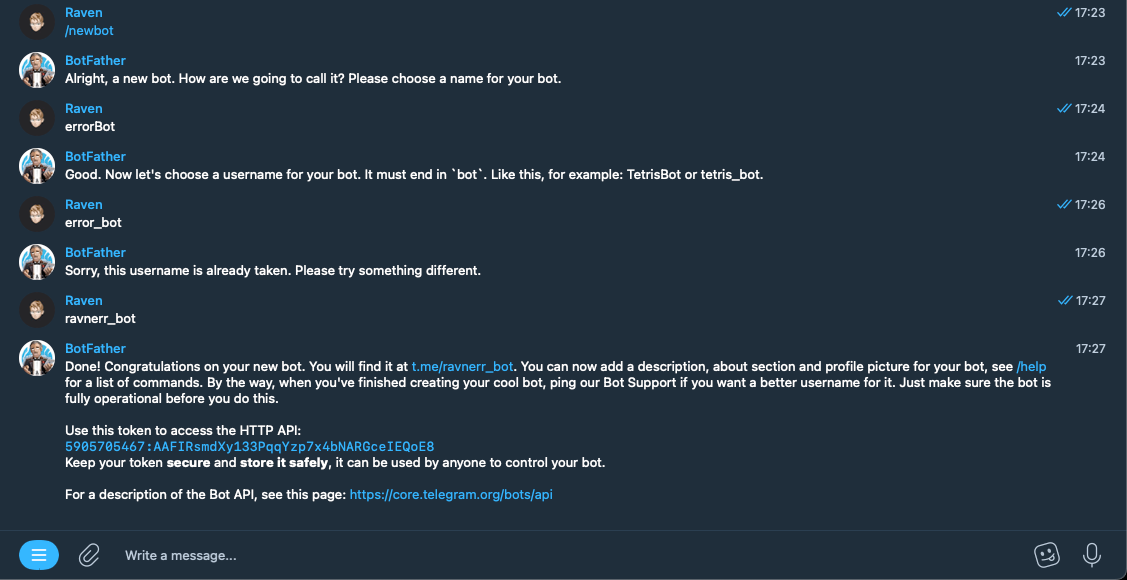
Identify our telegram ChatID.
To identify our chatID we are going to use help of another telegram bot called @myidbot. Lookup @myidbot account in telegram search and click ‘start’ for conversation. It should look similar like on image below:
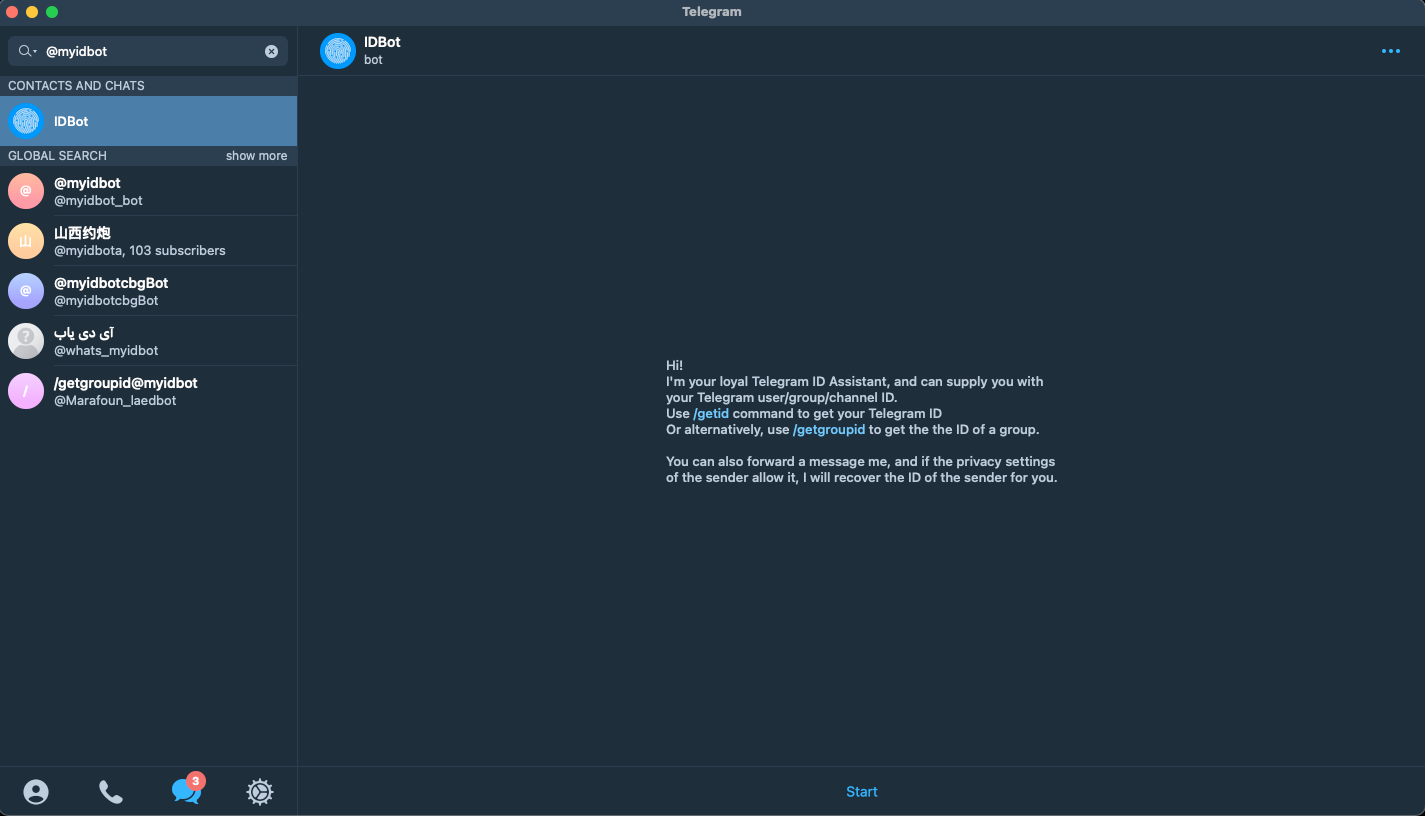
We are going to use another command “/getid”. Bot will answer us with our chatID. We will need this one in Python code.
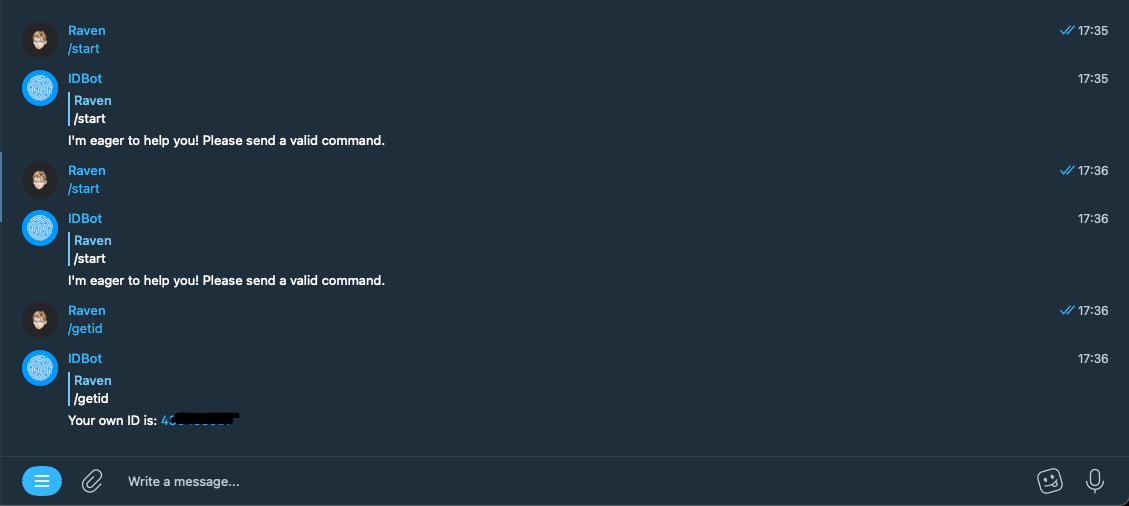
Write python code for sending messages.
Let's get started. I'm assuming you know the basics of Python, like using the requests library, setting up a virtual environment, and installing packages with Pip.
At first we need to install "requests" package using pip in our virtual environment.
pip install requests
Now we can create new python file. We are going to use our bot access token and chatid and requests library.
import requests
#BOT ACCESS TOKEN
BOT_API = '5763538887:AAGYefJ1jM_d18kVaXjhswFyzTnP_F35FrM'
#your CHATID number
CHAT_ID = '43XXXXXX'
def send_message(message):
apiURL = 'https://api.telegram.org/bot{apiToken}/sendMessage'
try:
response = requests.post(
apiURL.format(apiToken=BOT_API),
json={'chat_id': CHAT_ID, 'text': message
})
print(response.text)
except Exception as e:
print(e)
send_message('This is error from Django:\n This is test error message.')
Ok and that's all. You can try to test that. Happy error catching!